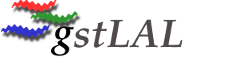 |
gstlal
0.8.1
|
3 # Copyright (C) 2010 Kipp Cannon, Chad Hanna, Leo Singer
5 # This program is free software; you can redistribute it and/or modify it
6 # under the terms of the GNU General Public License as published by the
7 # Free Software Foundation; either version 2 of the License, or (at your
8 # option) any later version.
10 # This program is distributed in the hope that it will be useful, but
11 # WITHOUT ANY WARRANTY; without even the implied warranty of
12 # MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General
13 # Public License for more details.
15 # You should have received a copy of the GNU General Public License along
16 # with this program; if not, write to the Free Software Foundation, Inc.,
17 # 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
18 """Estimate power spectra from LIGO frames or simulated h(t)."""
20 ## @file gstlal_reference_psd
21 # This program will measure a power spectral density from data; See gstlal_reference_psd for help and usage.
23 # This program will measure the PSD of data Its input is anything
24 # supported by datasource.py.
26 # #### Graph of the gsreamer pipeline
28 # Please see reference_psd.measure_psd()
32 # See datasource.append_options() for additional usage cases for datasource specific command line options
34 # -# Measure the PSD of frame data found in a file called frame.cache (hint you can make frame cache with lalapps_path2cache, e.g., `$ ls testframes